Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- pass
- 2020 카카오 인턴십
- 코드 리뷰
- Level 2
- 2019 KAKAO BLIND
- 코드리뷰
- 부스트코스
- Level 3
- SWEA
- 삼성 SW 역량 테스트
- 2020 KAKAO BLIND
- DP
- DFS
- level 1
- 백준
- 시뮬레이션
- c++
- 백트래킹
- Gold 5
- 월간 코드 챌린지
- 구현
- 그리디
- next_permutation
- Level 4
- 브루트포스
- 스택/큐
- 프로그래머스
- Web
- BFS
- Gold 4
Archives
- Today
- Total
Min:D's Devlog
[프로그래머스][2019 KAKAO BLIND][C++] 길 찾기 게임 본문
문제
프로그래머스 2019 KAKAO BLIND RECRUITMENT - 길 찾기 게임 (Level 3)
코딩테스트 연습 - 길 찾기 게임
[[5,3],[11,5],[13,3],[3,5],[6,1],[1,3],[8,6],[7,2],[2,2]] [[7,4,6,9,1,8,5,2,3],[9,6,5,8,1,4,3,2,7]]
programmers.co.kr
문제 풀이
접근 방식
이 문제는 2019 카카오 블라인드 공채 1차 코딩테스트 5번 문제로,
이진 트리를 만들어 전위 순회와 후위 순회를 수행하는 문제이다.
이진 트리를 만들기 위해 Node 구조체를 만들어 x, y, index, 왼쪽 노드, 오른쪽 노드를 저장할 수 있도록 하였고,
주어진 nodeinfo에 인덱스를 추가한 후,
y값 기준 내림차순, x값 기준 오름차순으로 정렬해주었다.
정렬된 nodeinfo 벡터를 Node를 활용하여 트리 구조로 저장해주었고,전위 순회, 후위 순회 함수를 만들어서 답을 도출하였다.
풀이 코드 - C++
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
struct Node {
int x, y, index;
Node *left;
Node *right;
};
bool cmp(vector<int> &a, vector<int> &b) {
if (a[1] == b[1])
return a[0] < b[0];
else
return a[1] > b[1];
}
// 전위 순회
vector<int> precircuit(const Node *node, vector<int> &pre) {
pre.push_back(node->index);
if (node->left != NULL)
precircuit(node->left, pre);
if (node->right != NULL)
precircuit(node->right, pre);
return pre;
}
// 후위 순회
vector<int> postcircuit(const Node *node, vector<int> &post) {
if (node->left != NULL)
postcircuit(node->left, post);
if (node->right != NULL)
postcircuit(node->right, post);
post.push_back(node->index);
return post;
}
vector<vector<int>> solution(vector<vector<int>> nodeinfo) {
// 인덱스 추가
for (int i = 0; i < nodeinfo.size(); i++)
nodeinfo[i].push_back(i + 1);
// y값 내림차순, x값 오름차순 정렬
sort(nodeinfo.begin(), nodeinfo.end(), cmp);
// 트리 저장
Node root = { nodeinfo[0][0], nodeinfo[0][1], nodeinfo[0][2], NULL, NULL };
Node * iter;
for (int i = 1; i < nodeinfo.size(); i++) {
iter = &root;
while (1) {
if (iter->x > nodeinfo[i][0]) {
if (iter->left == NULL) {
iter->left = new Node{nodeinfo[i][0], nodeinfo[i][1], nodeinfo[i][2], NULL, NULL};
break;
}
else
iter = iter->left;
}
else {
if (iter->right == NULL) {
iter->right = new Node{nodeinfo[i][0], nodeinfo[i][1], nodeinfo[i][2], NULL, NULL};
break;
}
else
iter = iter->right;
}
}
}
// 전위 순회, 후위 순회
vector<vector<int>> answer;
vector<int> pre;
vector<int> post;
answer.push_back(precircuit(&root, pre));
answer.push_back(postcircuit(&root, post));
return answer;
}
실행 결과
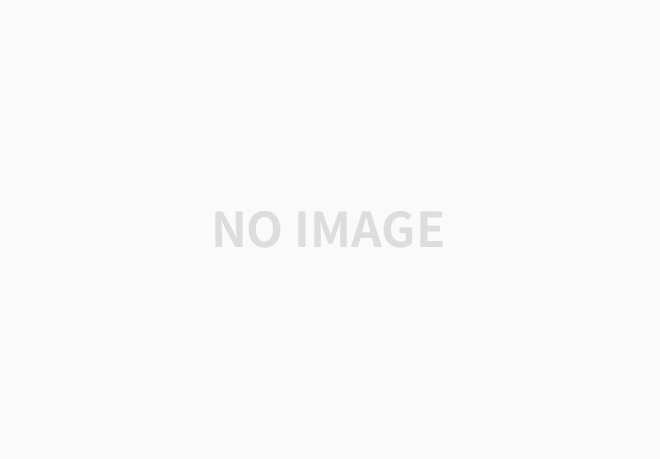
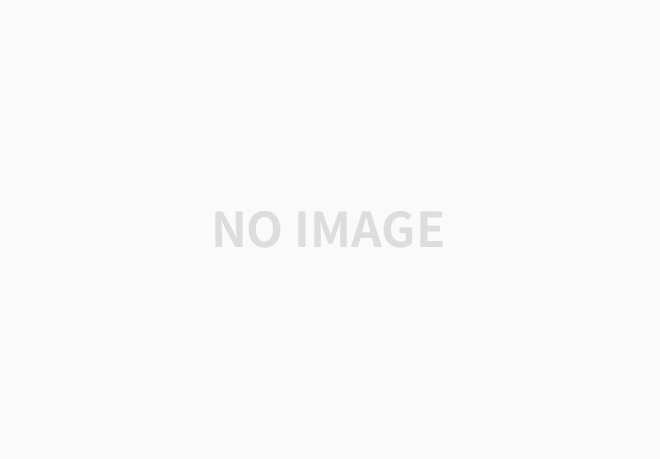
'알고리즘 > 프로그래머스' 카테고리의 다른 글
[프로그래머스][그리디][C++] 섬 연결하기 (0) | 2020.10.09 |
---|---|
[프로그래머스][DFS/BFS][C++] 타겟 넘버 (0) | 2020.09.24 |
[프로그래머스][월간 코드 챌린지 시즌 1][C++] 풍선 터트리기 (0) | 2020.09.22 |
[프로그래머스][월간 코드 챌린지 시즌 1][C++] 삼각 달팽이 (0) | 2020.09.21 |
[프로그래머스][월간 코드 챌린지 시즌 1][C++] 두개 뽑아서 더하기 (0) | 2020.09.19 |